Personal tools
swap_ranges

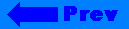
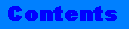
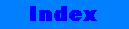
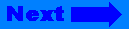
Click on the banner to return to the class reference home page.
swap_ranges
Algorithm
Summary
Exchange a range of values in one location with those in another
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
None
Synopsis
#include <algorithm> template <class ForwardIterator1, class ForwardIterator2> ForwardIterator2 swap_ranges (ForwardIterator1 first1, ForwardIterator1 last1, ForwardIterator2 first2);
Description
The swap_ranges algorithm exchanges corresponding values in two ranges, in the following manner:
For each non-negative integer n < (last - first) the function exchanges *(first1 + n) with *(first2 + n)). After completing all exchanges, swap_ranges returns an iterator that points to the end of the second container, i.e., first2 + (last1 -first1). The result of swap_ranges is undefined if the two ranges [first, last) and [first2, first2 + (last1 - first1)) overlap.
Example
// // swap.cpp // #include <vector> #include <algorithm> int main() { int d1[] = {6, 7, 8, 9, 10, 1, 2, 3, 4, 5}; // Set up a vector vector<int> v(d1,d1 + 10); // Output original vector cout << "For the vector: "; copy(v.begin(),v.end(),ostream_iterator<int,char>(cout," ")); // Swap the first five elements with the last five elements swap_ranges(v.begin(),v.begin()+5, v.begin()+5); // Output result cout << endl << endl << "Swapping the first five elements " << "with the last five gives: " << endl << " "; copy(v.begin(),v.end(),ostream_iterator<int,char>(cout," ")); return 0; } Output : For the vector: 6 7 8 9 10 1 2 3 4 5 Swapping the first five elements with the last five gives: 1 2 3 4 5 6 7 8 9 10 Swapping the first and last elements gives: 10 2 3 4 5 6 7 8 9 1
Warning
If your compiler does not support default template parameters, you need to always supply the Allocator template argument. For instance, you will need to write :
vector<int, allocator<int> >
instead of :
vector<int>
See Also
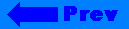
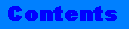
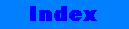
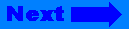
©Copyright 1996, Rogue Wave Software, Inc.