Personal tools
iter_swap

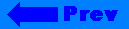
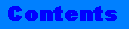
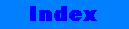
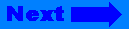
Click on the banner to return to the class reference home page.
iter_swap
Algorithm
Summary
Exchange values pointed at in two locations
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
None
Synopsis
#include <algorithm> template <class ForwardIterator1, class ForwardIterator2> void iter_swap (ForwardIterator1, ForwardIterator2);
Description
The iter_swap algorithm exchanges the values pointed at by the two iterators a and b.
Example
#include <vector> #include <algorithm> #include <iostream.h> int main () { int d1[] = {6, 7, 8, 9, 10, 1, 2, 3, 4, 5}; // // Set up a vector. // vector<int> v(d1+0, d1+10); // // Output original vector. // cout << "For the vector: "; copy(v.begin(), v.end(), ostream_iterator<int,char>(cout," ")); // // Swap the first five elements with the last five elements. // swap_ranges(v.begin(), v.begin()+5, v.begin()+5); // // Output result. // cout << endl << endl << "Swapping the first 5 elements with the last 5 gives: " << endl << " "; copy(v.begin(), v.end(), ostream_iterator<int,char>(cout," ")); // // Now an example of iter_swap -- swap first and last elements. // iter_swap(v.begin(), v.end()-1); // // Output result. // cout << endl << endl << "Swapping the first and last elements gives: " << endl << " "; copy(v.begin(), v.end(), ostream_iterator<int,char>(cout," ")); cout << endl; return 0; } Output : For the vector: 6 7 8 9 10 1 2 3 4 5 Swapping the first five elements with the last five gives: 1 2 3 4 5 6 7 8 9 10 Swapping the first and last elements gives: 10 2 3 4 5 6 7 8 9 1
Warning
If your compiler does not support default template parameters, then you will need to always supply the Allocator template argument. For instance, you'll have to write :
vector<int, allocator<int> >
instead of :
vector<int>
See Also
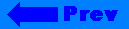
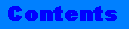
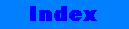
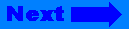
©Copyright 1996, Rogue Wave Software, Inc.