Personal tools
reverse

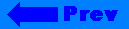
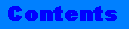
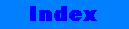
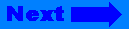
Click on the banner to return to the class reference home page.
reverse
Algorithm
- Summary
- Data Type and Member Function Indexes
- Synopsis
- Description
- Complexity
- Example
- Warning
- See Also
Summary
Reverse the order of elements in a collection.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
None
Synopsis
#include <algorithm> template <class BidirectionalIterator> void reverse (BidirectionalIterator first, BidirectionalIterator last);
Description
The algorithm reverse reverses the elements in a sequence so that the last element becomes the new first element, and the first element becomes the new last. For each non-negative integer i <= (last - first)/2 , reverse applies swap to all pairs of iterators first + I, (last - I) - 1.
Because the iterators are assumed to be bidirectional, reverse does not return anything.
Complexity
reverse performs exactly (last - first)/2 swaps.
Example
// // reverse.cpp // #include <algorithm> #include <vector> #include <iostream.h> int main() { //Initialize a vector with an array of ints int arr[10] = {1,2,3,4,5,6,7,8,9,10}; vector<int> v(arr, arr+10); //Print out elements in original (sorted) order cout << "Elements before reverse: " << endl << " "; copy(v.begin(),v.end(),ostream_iterator<int,char>(cout," ")); cout << endl << endl; //Reverse the ordering reverse(v.begin(), v.end()); //Print out the reversed elements cout << "Elements after reverse: " << endl << " "; copy(v.begin(),v.end(),ostream_iterator<int,char>(cout," ")); cout << endl; return 0; } Output : Elements before reverse: 1 2 3 4 5 6 7 8 9 10 Elements after reverse: 10 9 8 7 6 5 4 3 2 1 A reverse_copy to cout: 1 2 3 4 5 6 7 8 9 10
Warning
If your compiler does not support default template parameters, then you need to always supply the Allocator template argument. For instance, you will need to write :
vector<int, allocator<int> >
instead of :
vector<int>
See Also
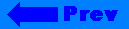
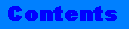
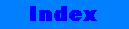
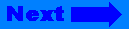
©Copyright 1996, Rogue Wave Software, Inc.