Personal tools
reverse_bidirectional_iterator, reverse_iterator

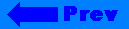
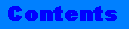
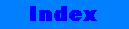
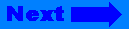
Click on the banner to return to the class reference home page.
reverse_bidirectional_iterator, reverse_iterator
Iterator
- Summary
- Data Type and Member Function Indexes
- Synopsis
- Description
- Complexity
- Interface
- Example
- Warning
- See Also
Summary
An iterator that traverses a collection backwards.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
None
Synopsis
#include <iterator> template <class BidirectionalIterator, class T, class Reference = T&, class Pointer = T* class Distance = ptrdiff_t> class reverse_bidirectional_iterator : public iterator<bidirectional_iterator_tag,T, Distance> ; template <class RandomAccessIterator, class T, class Reference = T&, class Pointer = T*, class Distance = ptrdiff_t> class reverse_iterator : public iterator<random_access_iterator_tag,T,Distance>;
Description
The iterators reverse_iterator and reverse_bidirectional_iterator correspond to random_access_iterator and bidirectional_iterator, except they traverse the collection they point to in the opposite direction. The fundamental relationship between a reverse iterator and its corresponding iterator i is established by the identity:
&*(reverse_iterator(i)) == &*(i-1);
This mapping is dictated by the fact that, while there is always a pointer past the end of a container, there might not be a valid pointer before its beginning.
The following are true for reverse_bidirectional_iterators :
These iterators may be instantiated with the default constructor or by a single argument constructor that initializes the new reverse_bidirectional_iterator with a bidirectional_iterator.
operator* returns a reference to the current value pointed to.
operator++ advances the iterator to the previous item (--current) and returns a reference to *this.
operator++(int) advances the iterator to the previous item (--current) and returns the old value of *this.
operator-- advances the iterator to the following item (++current) and returns a reference to *this.
operator--(int) Advances the iterator to the following item (++current) and returns the old value of *this.
operator== This non-member operator returns true if the iterators x and y point to the same item.
The following are true for reverse_iterators :
These iterators may be instantiated with the default constructor or by a single argument constructor which initializes the new reverse_iterator with a random_access_iterator.
operator* returns a reference to the current value pointed to.
operator++ advances the iterator to the previous item (--current) and returns a reference to *this.
operator++(int) advances the iterator to the previous item (--current) and returns the old value of *this.
operator-- advances the iterator to the following item (++current) and returns a reference to *this.
operator--(int) advances the iterator to the following item (++current) and returns the old value of *this.
operator== is a non-member operator returns true if the iterators x and y point to the same item.
operator!= is a non-member operator returns !(x==y).
operator< is a non-member operator returns true if the iterator x precedes the iterator y.
operator> is a non-member operator returns y < x.
operator<= is a non-member operator returns !(y < x).
operator>= is a non-member operator returns !(x < y).
The remaining operators (<, +, - , +=, -=) are redefined to behave exactly as they would in a random_access_iterator, except with the sense of direction reversed.
Complexity
All iterator operations are required to take at most amortized constant time.
Interface
template <class BidirectionalIterator, class T, class Reference = T&, class Pointer = T*, class Distance = ptrdiff_t> class reverse_bidirectional_iterator : public iterator<bidirectional_iterator_tag,T, Distance> { typedef reverse_bidirectional_iterator<BidirectionalIterator, T, Reference, Pointer, Distance> self; friend bool operator== (const self&, const self&); public: reverse_bidirectional_iterator (); explicit reverse_bidirectional_iterator (BidirectionalIterator); BidirectionalIterator base (); Reference operator* (); self& operator++ (); self operator++ (int); self& operator-- (); self operator-- (int); }; // Non-member Operators template <class BidirectionalIterator, class T, class Reference, class Pointer, class Distance> bool operator== ( const reverse_bidirectional_iterator <BidirectionalIterator,T,Reference,Pointer,Distance>&, const reverse_bidirectional_iterator <BidirectionalIterator,T,Reference,Pointer,Distance>&); template <class BidirectionalIterator, class T, class Reference, class Pointer, class Distance> bool operator!= ( const reverse_bidirectional_iterator <BidirectionalIterator,T,Reference,Pointer,Distance>&, const reverse_bidirectional_iterator <BidirectionalIterator,T,Reference,Pointer,Distance>&); template <class RandomAccessIterator, class T, class Reference = T&, class Pointer = T*, class Distance = ptrdiff_t> class reverse_iterator : public iterator<random_access_iterator_tag,T,Distance> { typedef reverse_iterator<RandomAccessIterator, T, Reference, Pointer, Distance> self; friend bool operator== (const self&, const self&); friend bool operator< (const self&, const self&); friend Distance operator- (const self&, const self&); friend self operator+ (Distance, const self&); public: reverse_iterator (); explicit reverse_iterator (RandomAccessIterator); RandomAccessIterator base (); Reference operator* (); self& operator++ (); self operator++ (int); self& operator-- (); self operator-- (int); self operator+ (Distance) const; self& operator+= (Distance); self operator- (Distance) const; self& operator-= (Distance); Reference operator[] (Distance); }; // Non-member Operators template <class RandomAccessIterator, class T, class Reference, class Pointer, class Distance> bool operator== ( const reverse_iterator<RandomAccessIterator, T, Reference, Pointer, Distance>&, const reverse_iterator<RandomAccessIterator, T, Reference, Pointer, Distance>&); template <class RandomAccessIterator, class T, class Reference, class Pointer, class Distance> bool operator!= ( const reverse_iterator<RandomAccessIterator, T, Reference, Pointer, Distance>&, const reverse_iterator<RandomAccessIterator, T, Reference, Pointer, Distance>&); template <class RandomAccessIterator, class T, class Reference, class Pointer, class Distance> bool operator< ( const reverse_iterator<RandomAccessIterator, T, Reference, Pointer, Distance>&, const reverse_iterator<RandomAccessIterator, T, Reference, Pointer, Distance>&); template <class RandomAccessIterator, class T, class Reference, class Pointer, class Distance> bool operator> ( const reverse_iterator<RandomAccessIterator, T, Reference, Pointer, Distance>&, const reverse_iterator<RandomAccessIterator, T, Reference, Pointer, Distance>&); template <class RandomAccessIterator, class T, class Reference, class Pointer, class Distance> bool operator<= ( const reverse_iterator<RandomAccessIterator, T, Reference, Pointer, Distance>&, const reverse_iterator<RandomAccessIterator, T, Reference, Pointer, Distance>&); template <class RandomAccessIterator, class T, class Reference, class Pointer, class Distance> bool operator>= ( const reverse_iterator<RandomAccessIterator, T, Reference, Pointer, Distance>&, const reverse_iterator<RandomAccessIterator, T, Reference, Pointer, Distance>&); template <class RandomAccessIterator, class T, class Reference, class Pointer, class Distance> Distance operator- ( const reverse_iterator<RandomAccessIterator, T, Reference, Pointer, Distance>&, const reverse_iterator<RandomAccessIterator, T, Reference, Pointer, Distance>&); template <class RandomAccessIterator, class T, class Reference, class Pointer, class Distance> reverse_iterator<RandomAccessIterator, T, Reference, Pointer, Distance> operator+ ( Distance, const reverse_iterator<RandomAccessIterator, T, Reference, Pointer, Distance>&);
Example
// // rev_itr.cpp // #include <iterator> #include <vector> #include <iostream.h> int main() { //Initialize a vector using an array int arr[4] = {3,4,7,8}; vector<int> v(arr,arr+4); //Output the original vector cout << "Traversing vector with iterator: " << endl << " "; for(vector<int>::iterator i = v.begin(); i != v.end(); i++) cout << *i << " "; //Declare the reverse_iterator vector<int>::reverse_iterator rev(v.end()); vector<int>::reverse_iterator rev_end(v.begin()); //Output the vector backwards cout << endl << endl; cout << "Same vector, same loop, reverse_itertor: " << endl << " "; for(; rev != rev_end; rev++) cout << *rev << " "; return 0; } Output : Traversing vector with iterator: 3 4 7 8 Same vector, same loop, reverse_itertor: 8 7 4 3
Warning
If your compiler does not support default template parameters, then you need to always supply the Allocator template argument. For instance, you will need to write :
vector<int, allocator<int> >
instead of :
vector<int>
See Also
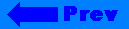
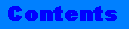
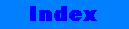
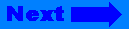
©Copyright 1996, Rogue Wave Software, Inc.