Personal tools
front_insert_iterator, front_inserter

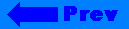
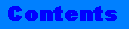
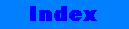
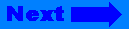
Click on the banner to return to the class reference home page.
front_insert_iterator, front_inserter
Insert Iterator
- Summary
- Data Type and Member Function Indexes
- Synopsis
- Description
- Interface
- Constructor
- Operators
- Non-member Function
- Example
- Warnings
- See Also
Summary
An insert iterator used to insert items at the beginning of a collection.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
Member Functions |
front_inserter() operator*() operator++() operator=() |
Synopsis
#include <iterator> template <class Container> class front_insert_iterator : public output_iterator ;
Description
Insert iterators let you insert new elements into a collection rather than copy a new element's value over the value of an existing element. The class front_insert_iterator is used to insert items at the beginning of a collection. The function front_inserter creates an instance of a front_insert_iterator for a particular collection type. A front_insert_iterator can be used with deques and lists, but not with maps or sets.
Note that a front_insert_iterator makes each element that it inserts the new front of the container. This has the effect of reversing the order of the inserted elements. For example, if you use a front_insert_iterator to insert "1" then "2" then "3" onto the front of container exmpl, you will find, after the three insertions, that the first three elements of exmpl are "3 2 1".
Interface
template <class Container> class front_insert_iterator : public output_iterator { public: explicit front_insert_iterator (Container&); front_insert_iterator<Container>& operator= (const typename Container::value_type&); front_insert_iterator<Container>& operator* (); front_insert_iterator<Container>& operator++ (); front_insert_iterator<Container> operator++ (int); }; template <class Container> front_insert_iterator<Container> front_inserter (Container&);
Constructor
explicit front_insert_iterator(Container& x);
Constructor. Creates an instance of a front_insert_iterator associated with container x.
Operators
front_insert_iterator<Container>& operator=(const typename Container::value_type& value);
Assignment Operator. Inserts a copy of value on the front of the container, and returns *this.
front_insert_iterator<Container>& operator*();
Returns *this (the input iterator itself).
front_insert_iterator<Container>& operator++();
front_insert_iterator<Container> operator++(int);
Increments the insert iterator and returns *this.
Non-member Function
template <class Container> front_insert_iterator<Container> front_inserter(Container& x)
Returns a front_insert_iterator that will insert elements at the beginning of container x. This function allows you to create front insert iterators inline.
Example
// // ins_itr.cpp // #include <iterator> #include <deque> #include <iostream.h> int main () { // // Initialize a deque using an array. // int arr[4] = { 3,4,7,8 }; deque<int> d(arr+0, arr+4); // // Output the original deque. // cout << "Start with a deque: " << endl << " "; copy(d.begin(), d.end(), ostream_iterator<int>(cout," ")); // // Insert into the middle. // insert_iterator<deque<int> > ins(d, d.begin()+2); *ins = 5; *ins = 6; // // Output the new deque. // cout << endl << endl; cout << "Use an insert_iterator: " << endl << " "; copy(d.begin(), d.end(), ostream_iterator<int>(cout," ")); // // A deque of four 1s. // deque<int> d2(4, 1); // // Insert d2 at front of d. // copy(d2.begin(), d2.end(), front_inserter(d)); // // Output the new deque. // cout << endl << endl; cout << "Use a front_inserter: " << endl << " "; copy(d.begin(), d.end(), ostream_iterator<int>(cout," ")); // // Insert d2 at back of d. // copy(d2.begin(), d2.end(), back_inserter(d)); // // Output the new deque. // cout << endl << endl; cout << "Use a back_inserter: " << endl << " "; copy(d.begin(), d.end(), ostream_iterator<int>(cout," ")); cout << endl; return 0; } Output : Start with a deque: 3 4 7 8 Use an insert_iterator: 3 4 5 6 7 8 Use a front_inserter: 1 1 1 1 3 4 5 6 7 8 Use a back_inserter: 1 1 1 1 3 4 5 6 7 8 1 1 1 1
Warnings
If your compiler does not support default template parameters then you need to always supply the Allocator template argument. For instance you'll have to write:
deque<int, allocator<int> >
instead of:
deque<int>
See Also
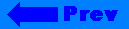
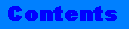
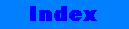
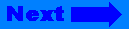
©Copyright 1996, Rogue Wave Software, Inc.