Personal tools
strstream

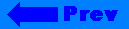
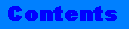
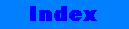
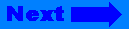
Click on the banner to return to the class reference home page.
strstream
basic_ostream strstream
basic_iostream
basic_ios
ios_base
basic_istream
- Data Type and Member Function Indexes
- Synopsis
- Description
- Interface
- Types
- Constructors
- Destructors
- Member Functions
- Examples
- See Also
- Standards Conformance
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
Synopsis
#include <strstream> class strstream : public basic_iostream<char>
Description
The class strstream provides functionality to read and write to an array in memory. It uses a private strstreambuf object to control the associated array. It inherits from basic_iostream<char> and therefore can use all the formatted and unformatted output and input functions.
Interface
class strstream : public basic_iostream<char> { public: typedef char_traits<char> traits; typedef char char_type; typedef typename traits::int_type int_type; typedef typename traits::pos_type pos_type; typedef typename traits::off_type off_type; strstream(); strstream(char *s, int n, ios_base::openmode = ios_base::out | ios_base::in); void freeze(int freezefl = 1); int pcount() const; virtual ~strstream(); strstreambuf *rdbuf() const; char *str(); };
Types
char_type
The type char_type is a synonym of type char.
int_type
The type int_type is a synonym of type traits::in_type.
off_type
The type off_type is a synonym of type traits::off_type.
pos_type
The type pos_type is a synonym of type traits::pos_type.
traits
The type traits is a synonym of type char_traits<char>.
Constructors
strstream();
Constructs an object of class strstream, initializing the base class basic_iostream<char> with the associated strstreambuf object. The strstreambuf object is initialized by calling its default constructor strstreambuf().
strstream(char* s,int n, ios_base::openmode mode = ios_base::out | ios_base::in);
if mode & app == 0 calls strstreambuf(s,n,s)
Otherwise calls strstreambuf(s,n,s + ::strlen(s))
Constructs an object of class strstream, initializing the base class basic_iostream<char> with the associated strstreambuf object. The strstreambuf object is initialized by calling one of two constructors:
Destructors
virtual ~strstream();
Destroys an object of class strstream.
Member Functions
void freeze(int freezefl = 1);
If freezefl is false, the function sets the freeze status to frozen.
Otherwise, it clears the freeze status.
If the mode is dynamic, alters the freeze status of the dynamic array object as follows:
int pcount() const;
Returns the size of the output sequence.
strstreambuf* rdbuf() const;
Returns a pointer to the strstreambuf object associated with the stream.
char* str();
Returns a pointer to the underlying array object which may be null.
Examples
// // stdlib/examples/manual/strstream.cpp // #include<strstream> void main ( ) { using namespace std; // create a bi-directional strstream object strstream inout; // output characters inout << "Das ist die rede von einem man" << endl; inout << "C'est l'histoire d'un home" << endl; inout << "This is the story of a man" << endl; char p[100]; // extract the first line inout.getline(p,100); // output the first line to stdout cout << endl << "Deutch :" << endl; cout << p; // extract the second line inout.getline(p,100); // output the second line to stdout cout << endl << "Francais :" << endl; cout << p; // extract the third line inout.getline(p,100); // output the third line to stdout cout << endl << "English :" << endl; cout << p; // output the all content of the // strstream object to stdout cout << endl << endl << inout.str(); }
See Also
char_traits(3C++), ios_base(3C++), basic_ios(3C++), strstreambuf(3C++), istrstream(3C++), ostrstream(3c++)
Working Paper for Draft Proposed International Standard for Information Systems--Programming Language C++, Annex D Compatibility features Section D.6.4
Standards Conformance
ANSI X3J16/ISO WG21 Joint C++ Committee
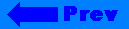
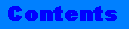
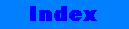
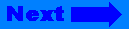
©Copyright 1996, Rogue Wave Software, Inc.