Personal tools
random_shuffle

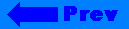
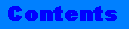
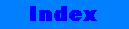
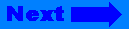
Click on the banner to return to the class reference home page.
random_shuffle
Algorithm
Summary
Randomly shuffles elements of a collection.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
None
Synopsis
#include <algorithm> template <class RandomAccessIterator> void random_shuffle (RandomAccessIterator first, RandomAccessIterator last); template <class RandomAccessIterator, class RandomNumberGenerator> void random_shuffle (RandomAccessIterator first, RandomAccessIterator last, RandomNumberGenerator& rand);
Description
The random_shuffle algorithm shuffles the elements in the range [first, last) with uniform distribution. random_shuffle can take a particular random number generating function object rand , where rand takes a positive argument n of distance type of the RandomAccessIterator and returns a randomly chosen value between 0 and n - 1.
Complexity
In the random_shuffle algorithm, (last - first) -1 swaps are done.
Example
// // rndshufl.cpp // #include <algorithm> #include <vector> #include <iostream.h> int main() { //Initialize a vector with an array of ints int arr[10] = {1,2,3,4,5,6,7,8,9,10}; vector<int> v(arr, arr+10); //Print out elements in original (sorted) order cout << "Elements before random_shuffle: " << endl << " "; copy(v.begin(),v.end(),ostream_iterator<int,char>(cout," ")); cout << endl << endl; //Mix them up with random_shuffle random_shuffle(v.begin(), v.end()); //Print out the mixed up elements cout << "Elements after random_shuffle: " << endl << " "; copy(v.begin(),v.end(),ostream_iterator<int,char>(cout," ")); cout << endl; return 0; } Output : Elements before random_shuffle: 1 2 3 4 5 6 7 8 9 10 Elements after random_shuffle: 7 9 10 3 2 5 4 8 1 6
Warning
If your compiler does not support default template parameters, you need to always supply the Allocator template argument. For instance, you will need to write :
vector<int, allocator<int> >
instead of :
vector<int>
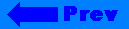
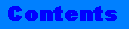
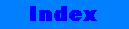
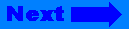
©Copyright 1996, Rogue Wave Software, Inc.