Personal tools
ostreambuf_iterator

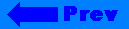
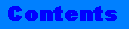
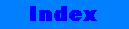
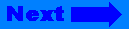
Click on the banner to return to the class reference home page.
ostreambuf_iterator
ostreambuf_iteratoroutput_iterator
- Data Type and Member Function Indexes
- Synopsis
- Description
- Interface
- Types
- Constructors
- Member Operators
- Public Member Function
- Examples
- See Also
- Standards Conformance
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
Data Types | |
char_type ostream_type streambuf_type traits_type |
Member Functions |
failed() operator*() operator++() operator=() |
Synopsis
#include <streambuf> template<class charT, class traits = char_traits<charT> > class ostreambuf_iterator : public output_iterator
Description
The template class ostreambuf_iterator writes successive characters onto the stream buffer object from which it was constructed. The operator= is used to write the characters and in case of failure the member function failed() returns true.
Interface
template<class charT, class traits = char_traits<charT> > class ostreambuf_iterator : public output_iterator { public: typedef charT char_type; typedef traits traits_type; typedef basic_streambuf<charT, traits> streambuf_type; typedef basic_ostream<charT, traits> ostream_type; ostreambuf_iterator(ostream_type& s) throw(); ostreambuf_iterator(streambuf_type *s) throw(); ostreambuf_iterator& operator*(); ostreambuf_iterator& operator++(); ostreambuf_iterator operator++(int); ostreambuf_iterator& operator=(charT c); bool failed( ) const throw(); };
Types
char_type
The type char_type is a synonym for the template parameter charT.
ostream_type
The type ostream_type is an instantiation of class basic_ostream on types charT and traits:
typedef basic_ostream<charT, traits> ostream_type;
streambuf_type
The type streambuf_type is an instantiation of class basic_streambuf on types charT and traits:
typedef basic_streambuf<charT, traits> streambuf_type;
traits_type
The type traits_type is a synonym for the template parameter traits.
Constructors
ostreambuf_iterator(ostream_type& s) throw();
Constructs an ostreambuf_iterator that uses the basic_streambuf object pointed at by s.rdbuf()to output characters. If s.rdbuf() is a null pointer, calls to the member function failed() return true.
ostreambuf_iterator(streambuf_type *s) throw();
Constructs an ostreambuf_iterator that uses the basic_streambuf object pointed at by s to output characters. If s is a null pointer, calls to the member function failed() return true.
Member Operators
ostreambuf_iterator& operator=(charT c);
Inserts the character c into the output sequence of the attached stream buffer. If the operation fails, calls to the member function failed() return true.
ostreambuf_iterator& operator++();
Returns *this.
ostreambuf_iterator operator++(int);
Returns *this.
ostreambuf_iterator operator*();
Returns *this.
Public Member Function
bool failed() const throw();
Returns true if the iterator failed inserting a characters or false otherwise.
Examples
// // stdlib/examples/manual/ostreambuf_iterator.cpp // #include<iostream> #include<fstream> void main ( ) { using namespace std; // create a filebuf object filebuf buf; // open the file iter_out and link it to the filebuf object buf.open("iter_out", ios_base::in | ios_base::out ); // create an ostreambuf_iterator and link it to // the filebuf object ostreambuf_iterator<char> out_iter(&buf); // output into the file using the ostreambuf_iterator for(char i=64; i<128; i++ ) out_iter = i; // seek to the beginning of the file buf.pubseekpos(0); // create an istreambuf_iterator and link it to // the filebuf object istreambuf_iterator<char> in_iter(&buf); // construct an end of stream iterator istreambuf_iterator<char> end_of_stream_iterator; cout << endl; // output the content of the file while( !in_iter.equal(end_of_stream_iterator) ) // use both operator++ and operator* cout << *in_iter++; cout << endl; }
See Also
basic_streambuf(3C++), basic_ostream(3C++), istreambuf_iterator(3C++)
Working Paper for Draft Proposed International Standard for Information Systems--Programming Language C++, Section 24.4.4
Standards Conformance
ANSI X3J16/ISO WG21 Joint C++ Committee
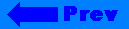
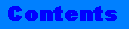
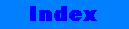
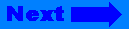
©Copyright 1996, Rogue Wave Software, Inc.