Personal tools
includes

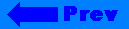
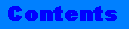
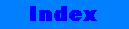
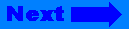
Click on the banner to return to the class reference home page.
includes
Algorithm
- Summary
- Data Type and Member Function Indexes
- Synopsis
- Description
- Complexity
- Example
- Warnings
- See Also
Summary
Basic set operation for sorted sequences.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
None
Synopsis
#include <algorithm> template <class InputIterator1, class InputIterator2> bool includes (InputIterator1 first1, InputIterator1 last1, InputIterator2 first2, InputIterator2 last2); template <class InputIterator1, class InputIterator2, class Compare> bool includes (InputIterator1 first1, InputIterator1 last1, InputIterator2 first2, InputIterator2 last2, Compare comp);
Description
The includes algorithm compares two sorted sequences and returns true if every element in the range [first2, last2) is contained in the range [first1, last1). It returns false otherwise. includes assumes that the sequences are sorted using the default comparison operator less than (<), unless an alternative comparison operator (comp) is provided.
Complexity
At most ((last1 - first1) + (last2 - first2)) * 2 -1 comparisons are performed.
Example
// // includes.cpp // #include <algorithm> #include <set> #include <iostream.h> int main() { //Initialize some sets int a1[10] = {1,2,3,4,5,6,7,8,9,10}; int a2[6] = {2,4,6,8,10,12}; int a3[4] = {3,5,7,8}; set<int, less<int> > all(a1, a1+10), even(a2, a2+6), small(a3,a3+4); //Demonstrate includes cout << "The set: "; copy(all.begin(),all.end(), ostream_iterator<int,char>(cout," ")); bool answer = includes(all.begin(), all.end(), small.begin(), small.end()); cout << endl << (answer ? "INCLUDES " : "DOES NOT INCLUDE "); copy(small.begin(),small.end(), ostream_iterator<int,char>(cout," ")); answer = includes(all.begin(), all.end(), even.begin(), even.end()); cout << ", and" << endl << (answer ? "INCLUDES" : "DOES NOT INCLUDE "); copy(even.begin(),even.end(), ostream_iterator<int,char>(cout," ")); cout << endl << endl; return 0; } Output : The set: 1 2 3 4 5 6 7 8 9 10 INCLUDES 3 5 7 8 , and DOES NOT INCLUDE 2 4 6 8 10 12
Warnings
If your compiler does not support default template parameters, then you need to always supply the Allocator template argument. For instance, you'll have to write :
set<int, less<int>, allocator<int> >
instead of
set<int>
See Also
set, set_union, set_intersection, set_difference, set_symmetric_difference
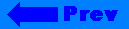
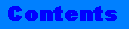
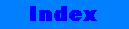
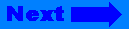
©Copyright 1996, Rogue Wave Software, Inc.