Personal tools
for_each

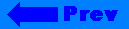
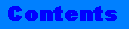
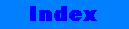
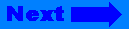
Click on the banner to return to the class reference home page.
for_each
Algorithm
- Summary
- Data Type and Member Function Indexes
- Synopsis
- Description
- Complexity
- Example
- Warning
- See Also
Summary
Applies a function to each element in a range.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
None
Synopsis
#include <algorithm> template <class InputIterator, class Function> void for_each(InputIterator first, InputIterator last, Function f);
Description
The for_each algorithm applies function f to all members of the sequence in the range [first, last), where first and last are iterators that define the sequence. Since this a non-mutating algorithm, the function f cannot make any modifications to the sequence, but it can achieve results through side effects (such as copying or printing). If f returns a result, the result is ignored.
Complexity
The function f is applied exactly last - first times.
Example
// // for_each.cpp // #include <vector> #include <algorithm> #include <iostream.h> // Function class that outputs its argument times x template <class Arg> class out_times_x : private unary_function<Arg,void> { private: Arg multiplier; public: out_times_x(const Arg& x) : multiplier(x) { } void operator()(const Arg& x) { cout << x * multiplier << " " << endl; } }; int main() { int sequence[5] = {1,2,3,4,5}; // Set up a vector vector<int> v(sequence,sequence + 5); // Setup a function object out_times_x<int> f2(2); for_each(v.begin(),v.end(),f2); // Apply function return 0; } Output : 2 4 6 8 10
Warning
If your compiler does not support default template parameters then you need to always supply the Allocator template argument. For instance you'll have to write:
vector<int, allocator<int> >
instead of:
vector<int>
See Also
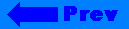
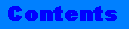
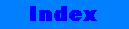
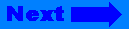
©Copyright 1996, Rogue Wave Software, Inc.