Personal tools
auto_ptr

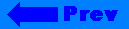
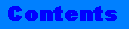
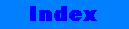
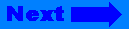
Click on the banner to return to the class reference home page.
auto_ptr
Memory Management
- Summary
- Data Type and Member Function Indexes
- Synopsis
- Description
- Interface
- Constructors and Destructors
- Operators
- Member Functions
- Example
Summary
A simple, smart pointer class.
Data Type and Member Function Indexes
(exclusive of constructors and destructors)
Member Functions |
get() operator*() ()">operator->() operator=() release() |
Synopsis
#include <memory> template <class X> class auto_ptr;
Description
The template class auto_ptr holds onto a pointer obtained via new and deletes that object when the auto_ptr object itself is destroyed (such as when leaving block scope). auto_ptr can be used to make calls to operator new exception-safe. The auto_ptr class provides semantics of strict ownership: an object may be safely pointed to by only one auto_ptr, so copying an auto_ptr copies the pointer and transfers ownership to the destination if the source had already had ownership.
Interface
template <class X> class auto_ptr { public: // constructor/copy/destroy explicit auto_ptr (X* = 0) throw(); template <class Y> auto_ptr (const auto_ptr<Y>&) throw(); template <class Y> void operator= (const auto_ptr<Y>&) throw(); ~auto_ptr (); // members X& operator* () const throw(); X* operator-> () const throw(); X* get () const throw(); X* release () throw(); };
Constructors and Destructors
explicit auto_ptr (X* p = 0);
Constructs an object of class auto_ptr<X>, initializing the held pointer to p, and acquiring ownership of that pointer. Requires that p points to an object of class X or a class derived from X for which delete p is defined and accessible, or that p is a null pointer.
template <class Y> auto_ptr (const auto_ptr<Y>& a);
Copy constructor. Constructs an object of class auto_ptr<X>, and copies the argument a to *this. If a owned the underlying pointer then *this becomes the new owner of that pointer.
~auto_ptr ();
Deletes the underlying pointer.
Operators
template <class Y> void operator= (const auto_ptr<Y>& a);
Assignment operator. Copies the argument a to *this. If *this becomes the new owner of the underlying pointer. If a owned the underlying pointer then *this becomes the new owner of that pointer. If *this already owned a pointer, then that pointer is deleted first.
X& operator* () const;
Returns a reference to the object to which the underlying pointer points.
X* operator-> () const;
Returns the underlying pointer.
Member Functions
X* get () const;
Returns the underlying pointer.
X* release();
Releases ownership of the underlying pointer. Returns that pointer.
Example
// // auto_ptr.cpp // #include <iostream.h> #include <memory> // // A simple structure. // struct X { X (int i = 0) : m_i(i) { } int get() const { return m_i; } int m_i; }; int main () { // // b will hold a pointer to an X. // auto_ptr<X> b(new X(12345)); // // a will now be the owner of the underlying pointer. // auto_ptr<X> a = b; // // Output the value contained by the underlying pointer. // cout << a->get() << endl; // // The pointer will be deleted when a is destroyed on // leaving scope. // return 0; } Output : 12345
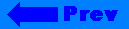
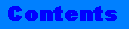
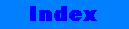
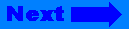
©Copyright 1996, Rogue Wave Software, Inc.